Selenium Java Pdf
Selenium Webdriver with Java (Basics + Advance + Architect) 4.3 (1,128 ratings) Course Ratings are calculated from individual students’ ratings and a variety of other signals, like age of rating and reliability, to ensure that they reflect course quality fairly and accurately.
Applitools SDKs works with existing test frameworks and simply takes screenshots of the page, element, region or an iframe and uploads them along with DOM snapshots to our Eyes server. Our AI then compares them with previous test executions' screenshots (aka Baselines) and tells if there is a bug or not. It's that simple!
# 1.1 Baseline vs. Checkpoint images
When you first run the test, our A.I. server simply stores those first set of screenshots as Baseline images. When you run the same test again (and everytime there after), the A.I. server will compare the new set of screenshots, aka Checkpoint images, with the corresponding Baseline images and higlights differences in pink color.
# 1.2 Marking the test as 'Pass' or 'Fail'
When the AI compares the baseline and the checkpoint image, if it finds a legitimate difference, it'll mark the test as Unresolved. This is because the AI doesn't know if the difference is because of a new feature or a real bug and will wait for you to manually mark it as a Pass/Fail for the 1st time.
If you mark the unresolved checkpoint image as a 'Fail', any further runs with similar difference will be automatically marked as 'Failed'.
If you mark the unresolved checkpoint image as a 'Pass', then it means that the difference is due to a new feature and so we update the new checkpoint image as the new baseline and mark the current test as Pass. And going forward we'll compare any future tests with this new baseline.
Java 4 Selenium Webdriver Pdf
Note:
Applitools AI has been trained with 100s of millions of images. It doesn't do a pixel-by-pixel comparison because it leads to a lot of false positives, but instead simulates real human eyes and ignore normal differences that humans would ignore and only highlight those that humans would highlight as bugs.
ACCURACY: A.I's current accuracy rate is 99.9999%! Which means for most applications that odds that you'll see false-positives is 1 in a million!
# A powerful test results dashboard
We provide a state-of-the-art dashboard that makes it very easy for you to analyze differences, report bugs straight from the dashboard and so on.
The following Gifs shows various tools Applitools provide to easily analyze various differences
# Highlight differences between the baseline and checkpoint
# Zoom into diffs
# Toggle differences between baseline and checkpoint
# Show both baseline and checkpoint side-by-side
3. 🐞 Reporting bugs (straight into Jira or Github)
You can simply select a section on the image and directly file a bug in Jira or Github. No need to manually take screenshots, write steps and explain things!Create a free Applitools account and get the Applitools API KEY
Have Java v10 or higher installed
Install Eclipse or IntelliJ editor
Install ChromeDriver on your machine and make sure it's is in the
PATH
.Here are some resources from the internet that'll help you.
Tip: On Mac, place the
chromedriver
executable in/usr/local/bin
folder so Eclipse and IntelliJ can find it.Install Maven. You can download the Maven binary Zip file from here.
- It should look something like
apache-maven-3.5.4-bin.zip
- Follow the installation instructions from here to add it to the
PATH
.
TIP
- It's better if you add it permanently to the environment so when you open a new Terminal the values will persist. Otherwise, you may have to redo it for everytime you open the Terminal. This means you should put it in the
~/.bash_profile
file (Mac) or in System variables in Windows. For more, see the Steps for addingChromedriver
to thePATH
below. - The Maven executable is inside
/bin
folder of the extracted Maven directory. So you must include/bin
. It should look something like:/Users/raja/apps/apache-maven-3.5.4/bin
# Test your Maven setup
- Make sure to restart the Terminal or Command line prompt to load the new environment variables.
- Run
mvn -v
. You should see something like below:
- It should look something like
Note:
Yoy may store the Applitools API Key in the environment variable. While this works for running the tests in the command line, if you are reading it from Eclipse or IntelliJ, then you need to launch those editors from the Terminal to read them. Otherwise, these editors won't load the environment variables!
Mac: export APPLITOOLS_API_KEY='YOUR_API_KEY'
Windows: set APPLITOOLS_API_KEY='YOUR_API_KEY'
- Get the code:
- Option 1:
git clone https://github.com/applitools/tutorial-selenium-java-basic.git
- Option 2: Download it as a Zip file and unzip it.
- Option 1:
- Import the folder as a Maven file in Eclipse or IntelliJ.
TIP
Make sure to set
APPLITOOLS_API_KEY
(see prerequisits section).Open Eclipse from Terminal so it can load the environment variables. On Mac, do something like:
open /Applications/Eclipse.app
❗️You may need to force download Maven dependencies
- In Eclipse:
- Right click on the main Project folder > Maven > Update Project
- Select 'Force Update of Snapshots/Releases' checkbox
- Press OK
- In Eclipse:
Change the Applitools API key in the
BasicDemo.java
. Get one by logging into Applitools > Person Icon > My API KeyRun the test
In Eclipse:
- Right click on the Project (or anywhere in the code) > Run As > JUnit Test
In Command line, run the following Maven command:
mvn -Dtest=BasicDemo test
After you run one set of tests, you now have the baselins. Run the same test but with this URL:
https://demo.applitools.com/index_v2.html
. This version of the demo app has some visual bugs so you can see how it all works. And the second set of screenshots are called 'Checkpoint images'.
# 1. Create a Maven project and add the following dependencies to the pom.xml
# 2. Import the SDK
# 3. Initialize the SDK
# 4. Start the test
# 5. Add eyes.checkWindow to take a screenshot
Every checkWindow
or (similar methods such as checkRegion
, checkElement
, checkFrame
) take a screenshot and upload it to the server for analysis. And since they are part of the test, each screenshot essentially becomes a test step. Note that you can add as many check
statements as you need after you open the tests.
# 6. End test
# 7. Get the test results
In real world testing, you'll need to test your app on multiple browsers, viewports and mobile devices. So you'll need to run and re-run ALL the tests in different browsers and multiple viewports to ensure things are fine - which could take hours and hours to complete. You may also encounter browsers not opening properly, or hanging and what not.
What if you could run in just one browser, just once, and still do cross-browser tests across various browsers, viewports and multiple mobile emulators? That's where Visual Grid [BETA] comes in.
TL;DR
When you execute this tutorial, it'll run the test locally in Chrome browser but will show results in the following 5 combinations:
- Chrome browser (800 X 600 viewport)
- Chrome browser (700 X 500 viewport)
- Firefox browser (1200 X 800 viewport)
- Firefox browser (1600 X 1200 viewport)
- An iPhone4 emulator

... all in ~30 seconds!⏰🚀
Sounds interesting?
Go through our Visual Grid tutorial!🚀
# Resources
- File a Ticket.
Terms & ConditionsPrivacy PolicyGDPR© 2019 Applitools. All rights reserved.
In Selenium automation, if the elements are not found by the general locators like id, class, name, etc. then XPath is used to find an element on the web page .
In this tutorial, we will learn about the xpath and different XPath expression to find the complex or dynamic elements, whose attributes changes dynamically on refresh or any operations.
In this tutorial, you will learn-
What is XPath?
XPath is defined as XML path. It is a syntax or language for finding any element on the web page using XML path expression. XPath is used to find the location of any element on a webpage using HTML DOM structure. The basic format of XPath is explained below with screen shot.
Syntax for XPath:
XPath contains the path of the element situated at the web page. Standard syntax for creating XPath is.
- // : Select current node.
- Tagname: Tagname of the particular node.
- @: Select attribute.
- Attribute: Attribute name of the node.
- Value: Value of the attribute.
XPath Locators | Find different elements on web page |
ID | To find the element by ID of the element |
Classname | To find the element by Classname of the element |
Name | To find the element by name of the element |
Link text | To find the element by text of the link |
XPath | XPath required for finding the dynamic element and traverse between various elements of the web page |
CSS path | CSS path also locates elements having no name, class or ID. |
Types of X-path
There are two types of XPath:
1) Absolute XPath
2) Relative XPath
Absolute XPath:
It is the direct way to find the element, but the disadvantage of the absolute XPath is that if there are any changes made in the path of the element then that XPath gets failed.
The key characteristic of XPath is that it begins with the single forward slash(/) ,which means you can select the element from the root node.
Below is the example of an absolute xpath expression of the element shown in the below screen.
Absolute xpath:
Relative xpath:
For Relative Xpath the path starts from the middle of the HTML DOM structure. It starts with the double forward slash (//), which means it can search the element anywhere at the webpage.
You can start from the middle of the HTML DOM structure and no need to write long xpath.
Below is the example of a relative XPath expression of the same element shown in the below screen. This is the common format used to find element through a relative XPath.
What are XPath axes.
XPath axes search different nodes in XML document from current context node. XPath Axes are the methods used to find dynamic elements, which otherwise not possible by normal XPath method having no ID , Classname, Name, etc.
Axes methods are used to find those elements, which dynamically change on refresh or any other operations. There are few axes methods commonly used in Selenium Webdriver like child, parent, ancestor, sibling, preceding, self, etc.
Selenium Cheat Sheet Java Pdf
Using XPath Handling complex & Dynamic elements in Selenium
1) Basic XPath:
XPath expression select nodes or list of nodes on the basis of attributes like ID , Name, Classname, etc. from the XML document as illustrated below.
Here is a link to access the page http://demo.guru99.com/v1/
Some more basic xpath expressions:
2) Contains():
Contains() is a method used in XPath expression. It is used when the value of any attribute changes dynamically, for example, login information.
The contain feature has an ability to find the element with partial text as shown in below example.
In this example, we tried to identify the element by just using partial text value of the attribute. In the below XPath expression partial value 'sub' is used in place of submit button. It can be observed that the element is found successfully.
Complete value of 'Type' is 'submit' but using only partial value 'sub'.
Complete value of 'name' is 'btnLogin' but using only partial value 'btn'.
In the above expression, we have taken the 'name' as an attribute and 'btn' as an partial value as shown in the below screenshot. This will find 2 elements (LOGIN & RESET) as their 'name' attribute begins with 'btn'.
Similarly, in the below expression, we have taken the 'id' as an attribute and 'message' as a partial value. This will find 2 elements ('User-ID must not be blank' & 'Password must not be blank') as its 'name' attribute begins with 'message'.
In the below expression, we have taken the 'text' of the link as an attribute and 'here' as a partial value as shown in the below screenshot. This will find the link ('here') as it displays the text 'here'.
3) Using OR & AND:
In OR expression, two conditions are used, whether 1st condition OR 2nd condition should be true. It is also applicable if any one condition is true or maybe both. Means any one condition should be true to find the element.
In the below XPath expression, it identifies the elements whose single or both conditions are true.
Highlighting both elements as 'LOGIN ' element having attribute 'type' and 'RESET' element having attribute 'name'.
In AND expression, two conditions are used, both conditions should be true to find the element. It fails to find element if any one condition is false.
In below expression, highlighting 'LOGIN' element as it having both attribute 'type' and 'name'.
4) Start-with function:
Start-with function finds the element whose attribute value
changes on refresh or any operation on the webpage.In this expression, match the starting text of the attribute is used to find the element whose attribute changes dynamically. You can also find the element whose attribute value is static (not changes).
For example -: Suppose the ID of particular element changes dynamically like:
Id=' message12'
Id=' message345'
Id=' message8769'
and so on.. but the initial text is same. In this case, we use Start-with expression.
In the below expression, there are two elements with an id starting 'message'(i.e., 'User-ID must not be blank' & 'Password must not be blank'). In below example, XPath finds those element whose 'ID' starting with 'message'.
5) Text():
In this expression, with text function, we find the element with exact text match as shown below. In our case, we find the element with text 'UserID'.
6) XPath axes methods:
These XPath axes methods are used to find the complex or dynamic elements. Below we will see some of these methods.
For illustrating these XPath axes method, we will use the Guru99 bank demo site.
a) Following:
Selects all elements in the document of the current node( ) [ UserID input box is the current node] as shown in the below screen.
There are 3 'input' nodes matching by using 'following' axis- password, login and reset button. If you want to focus on any particular element then you can use the below XPath method:
You can change the XPath according to the requirement by putting [1],[2]…………and so on.
With the input as '1', the below screen shot finds the particular node that is 'Password' input box element.
b) Ancestor:
Selenium Webdriver Documentation Java Pdf
The ancestor axis selects all ancestors element (grandparent, parent, etc.) of the current node as shown in the below screen.
In the below expression, we are finding ancestors element of the current node('ENTERPRISE TESTING' node).
There are 13 'div' nodes matching by using 'ancestor' axis. If you want to focus on any particular element then you can use the below XPath, where you change the number 1, 2 as per your requirement:
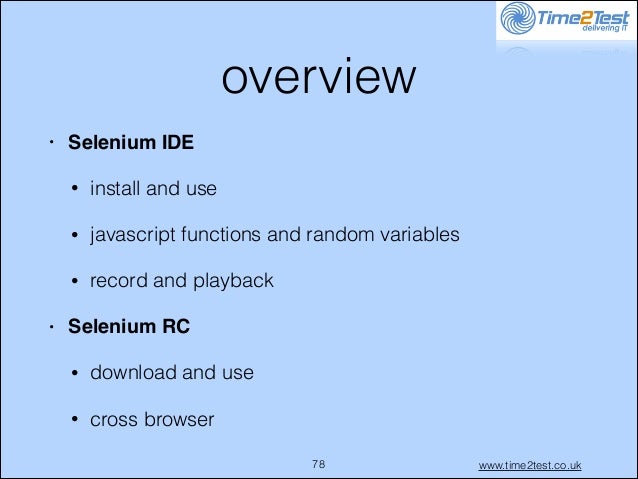
You can change the XPath according to the requirement by putting [1], [2]…………and so on.
c) Child:
Selects all children elements of the current node (Java) as shown in the below screen.
There are 71 'li' nodes matching by using 'child' axis. If you want to focus on any particular element then you can use the below xpath:
You can change the xpath according to the requirement by putting [1],[2]…………and so on.
d) Preceding:
Select all nodes that come before the current node as shown in the below screen.
In the below expression, it identifies all the input elements before 'LOGIN' button that is Userid and password input element.
There are 2 'input' nodes matching by using 'preceding' axis. If you want to focus on any particular element then you can use the below XPath:
You can change the xpath according to the requirement by putting [1],[2]…………and so on.
e) Following-sibling:
Select the following siblings of the context node. Siblings are at the same level of the current node as shown in the below screen. It will find the element after the current node.
One input nodes matching by using 'following-sibling' axis.
f) Parent:
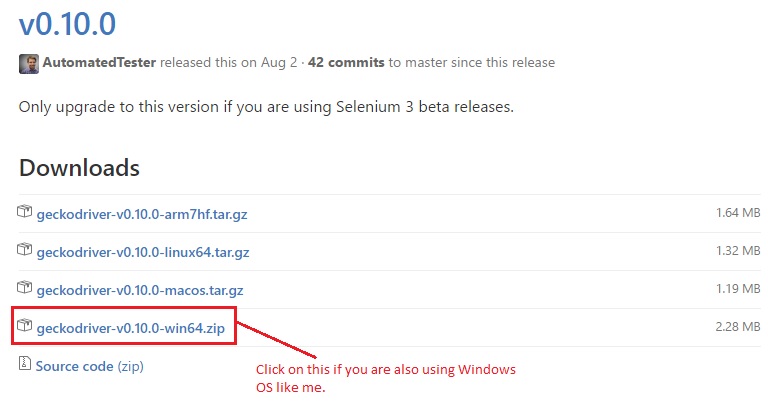
Selects the parent of the current node as shown in the below screen.
There are 65 'div' nodes matching by using 'parent' axis. If you want to focus on any particular element then you can use the below XPath:
You can change the XPath according to the requirement by putting [1],[2]…………and so on.
g) Self:
Selects the current node or 'self' means it indicates the node itself as shown in the below screen.
One node matching by using 'self ' axis. It always finds only one node as it represents self-element.
h) Descendant:
Selects the descendants of the current node as shown in the below screen.In the below expression, it identifies all the element descendants to current element ( 'Main body surround' frame element) which means down under the node (child node , grandchild node, etc.).
Selenium Java Example
There are 12 'link' nodes matching by using 'descendant' axis. If you want to focus on any particular element then you can use the below XPath:
You can change the XPath according to the requirement by putting [1],[2]…………and so on.
Summary:
Selenium Using Java
XPath is required to find an element on the web page as to do an operation on that particular element.
- There are two types of XPath:
- Absolute XPath
- Relative XPath
- XPath Axes are the methods used to find dynamic elements, which otherwise not possible to find by normal XPath method
- XPath expression select nodes or list of nodes on the basis of attributes like ID , Name, Classname, etc. from the XML document .